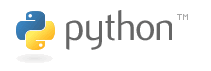
Install Python. It's a standard Windows installer so that's just point and click. Then make sure the path to 'python.exe' is in your PATH environment variable.
Install Apache using XAMPP. This is also available as Windows installer and you can also install MySQL, FileZilla, Tomcat and Mercury. I'd advice to install at least MySQL. After all a database is one of the minimal requirements for most websites. In my example XAMPP is installed to D:/Xampp.
Using the XAMPP control panel, start Apache and make sure it's running: open a browser and type 'localhost' as the URL. If it works it will show the XAMPP configuration screen.
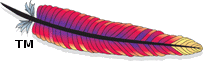
Now get the 'wsgi_mod.so' binary that matches your configuration. It must be the 32 bit or 64 bit version depending on system AND it must match the Apache version that is installed. Now XAMPP does not really show you which version it is using so the easiest way to find out is looking at the CHANGES.txt file in the apache folder. The current version of XAMPP (June 2013) contains version 2.4.3. This means that none of the 'wsgi_mod.so' files on the google.code page will work since they all target version 2.2 !
I Googled for 'mod_wsgi apache 2.4' and found it in the Apache Lounge .
Copy the 'wsgi_mod.so' to the 'apache/modules/' folder.
Add this line to 'httpd.conf' to load the mod_wsgi module:
LoadModule wsgi_module modules/mod_wsgi.so
Stop and start Apache to make sure it's still working.
Create a directory D:/Xampp/wsgi. Make sure you can run python scripts in this directory: open a command window, make sure you're in this directory and just type 'python'. If all is well it will show the installed python version and a command prompt. If not, check the environment variable 'PATH' and make sure the python folder is included.
Add the following lines to 'httpd.conf'. This will allow access to the wsgi directory, and make sure all wsgi requests are routed to the directory:
<Directory "D:/xampp/wsgi" >
AllowOverride None
Options None
Require all granted
</Directory>
# Python WSGI interface module
<IfModule wsgi_module>
WSGIPythonHome "D:/Python27"
WSGIScriptAlias / D:/xampp/wsgi
</IfModule>
Now create a text file with the following contents:
def application(environ, start_response):
status = '200 OK'
output = 'Hello World!'
response_headers = [('Content-type', 'text/plain'),
('Content-Length', str(len(output)))]
start_response(status, response_headers)
return [output]
Save it as 'hello_world.wsgi' in the 'wsgi' directory
Open your browser and type 'localhost/hello_world.wsgi'. And yes: It will show 'Hello World' !
Note that strictly spoken the
'WSGIPythonHome "D:/Python27"'
is not required for this example, but if you want to add code that uses the 'import' statement Apache needs to know where to find your Python directory.